Examples¶
Load the package into your python environment using the following command
from pybmrb import Spectra, Histogram
Spectra simulation¶
PyBMRB combines the chemical shift information from BMRB entries or NMR-STAR files using certain rules defined by the experiment type. In this method it can generate 1 H - 15 N - HSQC, 1 H - 13 C -HSQC and 1 H - 1 H-TOCSY. You may define which atom to be on X axis and which atom to be on Y axis to generate a generic 2D correlation spectrum.
1 H - 15 N - HSQC peak position simulation¶
Example 1: Single entry from BMRB
peak_list=Spectra.n15hsqc(bmrb_ids=15060, legend='residue')
Example 2: Multiple entries from BMRB along with a NMR-STAR file.
For multiple data set use data set as legend, so that you may turn on and off different data set. You may also use residue as legend to turn on and off different residue types
peak_list=Spectra.n15hsqc(bmrb_ids=[17076,17077], input_file_names='test_data/MyData.str', legend='dataset')
Example 3: Multiple entries from BMRB along with a NMR-STAR file and a peak list in csv format
peak_list=Spectra.n15hsqc(bmrb_ids=[17076,17077], input_file_names='test_data/MyData.str', peak_list='test_data/my_peak_list.csv', legend='dataset')
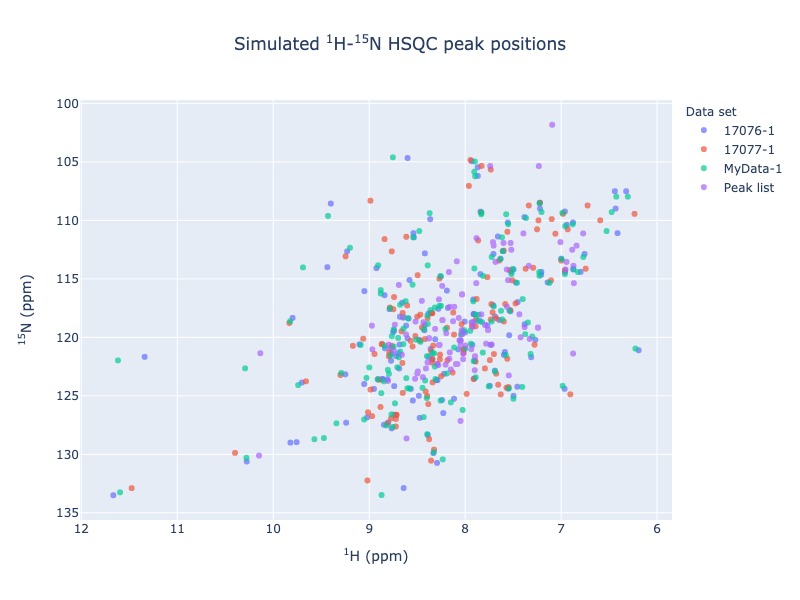
Click here for interactive html for n15-HSQC from BMRB entries along with NMR-STAR file and a peak list¶
Example 4: Multiple entries from BMRB with chemical shift tracking
peak_list=Spectra.n15hsqc(bmrb_ids=[17076,17077], input_file_names='test_data/MyData.str', legend='dataset', draw_trace=True)
1 H - 13 C - HSQC peak position simulation¶
Example 5: Single entry from BMRB
peak_list=Spectra.c13hsqc(bmrb_ids=15060, legend='residue')
Example 6: Multiple entries from BMRB
peak_list=Spectra.c13hsqc(bmrb_ids=[17074,17076,17077], legend='dataset')
Example 7: Multiple entries from BMRB with chemical shift tracking
peak_list=Spectra.c13hsqc(bmrb_ids=[17074,17076,17077], legend='dataset', draw_trace=True)
1 H - 1 H - TOCSY peak position simulation¶
Example 8: Single entry from BMRB
peak_list=Spectra.tocsy(bmrb_ids=15060, legend='residue')
Example 9: Multiple entries from BMRB
peak_list=Spectra.tocsy(bmrb_ids=[17074,17076,17077], legend='dataset')
Example 10: Multiple entries from BMRB with residues as legend
peak_list=Spectra.tocsy(bmrb_ids=[17074,17076,17077], legend='residue')
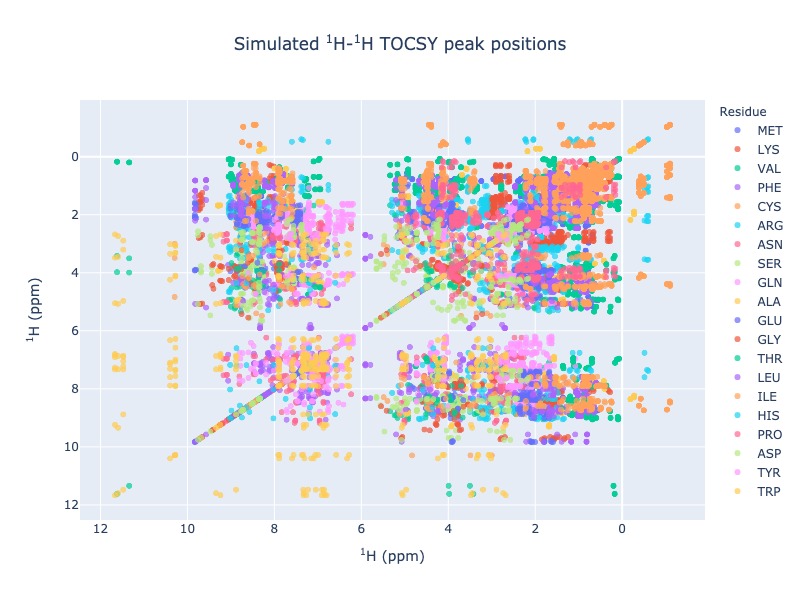
Click here for interactive html for TOCSY from BMRB entries 17074,17076 and 17076 with residues as legend¶
Example 11 : Multiple entries from BMRB with chemical shift tracking
peak_list=Spectra.tocsy(bmrb_ids=[17074,17076,17077], legend='dataset', draw_trace=True)
Please not the above TOCSY with chemical shift visualization will take some time to load, because of hundreds of traces
Generic 2D peak position simulation¶
You may use any two atoms in a residue to generate a generic 2D spectrum. For the following examples, N chemical shifts were used as x axis and CB chemical shifts were was used a Y axis.
Example 12: Single entry from BMRB
peak_list=Spectra.generic_2d(bmrb_ids=15060, atom_x='N', atom_y='CB', legend='residue')
Example 13: Multiple entries from BMRB
peak_list=Spectra.generic_2d(bmrb_ids=[17074,17076,17077], atom_x='N', atom_y='CB', legend='dataset')
Example 14: Multiple entries from BMRB with chemical shift tracking
peak_list=Spectra.generic_2d(bmrb_ids=[17074,17076,17077], atom_x='N', atom_y='CB', legend='dataset', draw_trace=True)
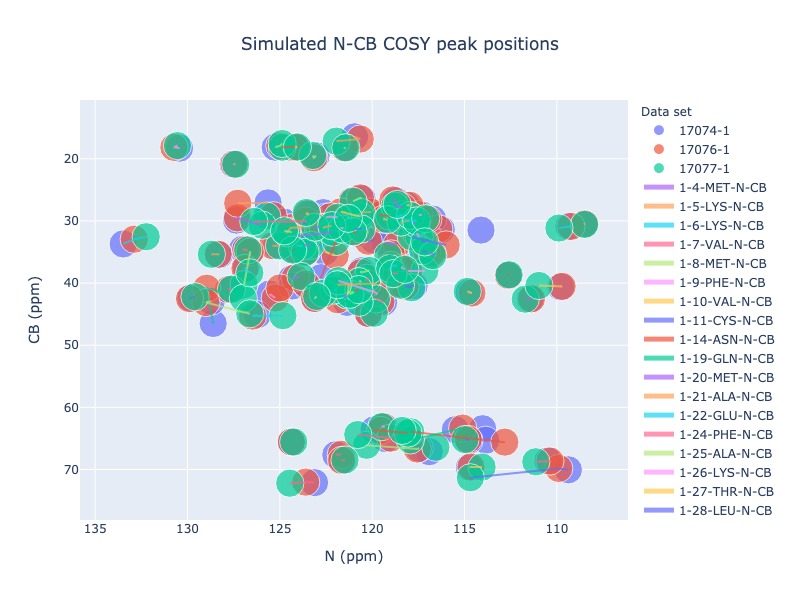
Click here for interactive html for generic 2D spectrum from BMRB entries 17074,17076 and 17076 with trace¶
Example 15: Include chemical shifts from preceding residue in the generic 2d spectrum
You may also include chemical shift from i-1 atom in the generic 2D spectrum, which will be shown using different size
peak_list = Spectra.generic_2d((bmrb_ids=15000, atom_x='N', atom_y='CA', include_preceding=True, legend='residue')
Example 16: Draw trace to show the walk along the sequence
You may draw a trace connecting i-1 to i to next i-1 and so on as long as the sequence is continuous. If you have missing chemical shift, then the trace will start from next possible residue
peak_list = Spectra.generic_2d((bmrb_ids=15000, atom_x='N', atom_y='CA', include_preceding=True, legend='residue', seq_walk=True)
Example 17: Include chemical shift from next residues in the generic 2d spectrum
You may also include chemical shift from i+1 atoms in the generic 2D spectrum, which will be shown using different size
peak_list = Spectra.generic_2d((bmrb_ids=15000, atom_x='N', atom_y='CA', include_next=True, legend='residue')
Example 18: Draw trace to show the walk along the sequence
You may draw a trace connecting i+1 to i to next i+1 and so on as long as the sequence is continuous. If you have missing chemical shift, then the trace will start from next possible residue
peak_list = Spectra.generic_2d((bmrb_ids=15000, atom_x='N', atom_y='CA', include_next=True, legend='residue', seq_walk=True)
Example 19: Draw trace to show the walk along the sequence ignoring missing residues
you may also draw traces from starting residue to end residue ignoring the missing ones
peak_list = Spectra.generic_2d((bmrb_ids=15000, atom_x='N', atom_y='CA', include_next=True, legend='residue', full_walk = True)
Chemical shift Histograms¶
PyBMRB is able to fetch database wide chemical shift data and plot the distribution in different ways. The default distribution would be Chemical shift vs number of instances(count). You may also plot the ‘percent’ or ‘probability’ or ‘probability density’ by providing desired value for ‘histnorm’. These distributions can be filtered using temperature range and PH range. Here are some of the examples.
Single distribution¶
Example 20: Chemical shift distribution of CYS-CB
cs_data=Histogram.hist(residue='CYS', atom='CB')
Example 21: Chemical shift distribution of CYS-CB with standard deviation cs_filt
You may exclude extreme values by using the cs_filt based on standard deviation. sd_limit=5 would exclude the values beyond 5 times standard deviation on moth sides of the mean
cs_data=Histogram.hist(residue='CYS', atom='CB', sd_limit=5 )
Example 22: Chemical shift distribution of CYS-CB with Ph cs_filt
You may use experimental conditions like Ph or temperature values as a cs_filt
cs_data=Histogram.hist(residue='CYS', atom='CB', sd_limit=5, ph_min=7.0, ph_max=8.2)
Example 23: Chemical shift distribution of CYS-CB as box plot
Box plot and Violin plot will show all the statistical properties of the distribution, when you mouse over the distribution.
cs_data=Histogram.hist(residue='CYS', atom='CB', plot_type='box')
Example 24: Chemical shift distribution of CYS-CB as violin plot
Box plot and Violin plot will show all the statistical properties of the distribution, when you mouse over the distribution.
cs_data=Histogram.hist(residue='CYS', atom='CB', plot_type='violin')
Multiple distribution¶
Example 25: Histogram from list of atoms
You may also provide list of atoms as input
cs_data=Histogram.hist(list_of_atoms=['GLN-CB','CYS-CB','TYR-CB'], histnorm='probability density')
Example 26: Violin plot for list of atoms
cs_data=Histogram.hist(list_of_atoms=['GLN-CB','CYS-CB','TYR-CB'], plot_type='violin')
Example 27: Histogram method supports wildcard
If you want to see the chemical shift distribution of protons in GLN, then you may use the following command. You may chose histnorm as ‘probability density’ to compare distributions
cs_data=Histogram.hist(residue='GLN', atom='H*', histnorm='probability density')
Example 28: Distribution of all atoms from a residue
If you want to see the chemical shift distribution of all atoms from a residue you may use atom=’*’ or simply leave out atom.
cs_data=Histogram.hist(residue='ASP', atom='*')
or
cs_data=Histogram.hist(residue='ASP')
Example 29: Distribution of specific atom type from al residues
If you want to see the chemical shift distribution CG atoms from all 20 standard residues you may use residue=*’ or simply leave out residue.
cs_data=Histogram.hist(residue='*', atom='CG*', histnorm='percent')
or
cs_data=Histogram.hist(atom='CG*', histnorm='percent')
2D Histograms¶
Example 30: Chemical shift correlation as 2d heatmap
cs_data=Histogram.hist2d(residue='CYS', atom1='CA', atom2='CB', sd_limit=5)
Example 31: Chemical shift correlation as contour heatmap
cs_data=Histogram.hist2d(residue='GLN', atom1='HE21', atom2='HE22', sd_limit=5, plot_type='contour')
Conditional histogram¶
Example 32: Conditional histogram with chemical shift filtering
You may cs_filt the chemical shift distribution of an atom in a residue based on the chemical shift values of one or more atom in the same residue. In the following example CYS-CB values are filtered based on CYS-H=8.9. During the seach 0.1ppm tolerance for protons and 2.0 ppm tolerance for heavy atoms is used.
cs_data=Histogram.conditional_hist(residue='CYS', atom='CB', histnorm='percent', filtering_rules=[('H',8.9)])
Example 33: Conditional histogram with chemical shift list
cs_data=Histogram.conditional_hist(residue='CYS', atom='CB', histnorm='percent', filtering_rules=[('H', 8.9), ('CA', 61)])
Data manipulation¶
If you are interested only in data manipulation please refer ChemicalShift and ChemicalShiftStatistics modules in Modules documentation.